I'm Mihiran Rupasinghe. And today we are going to look at a very important part in android. That is how to use web services in android.
First of all, I have to tell you, there is no any hard part here.. Its very simple.
In here, I'm going to use ASP.NET web service in Microsoft Visual Studio 2008 and Microsoft SQL server 2008. I'm going to show you how it works with remote database that can be accessed from anywhere in the world.
So lets get started !
First I created a database called SolutioDude. After that I created a table called member and inserted some values shown as below.
Ok. Now open visual studio and select File > New > Web site. And choose ASP.NET Web Service. Give a path where you can store the web service, select the language as Visual C# and press OK.
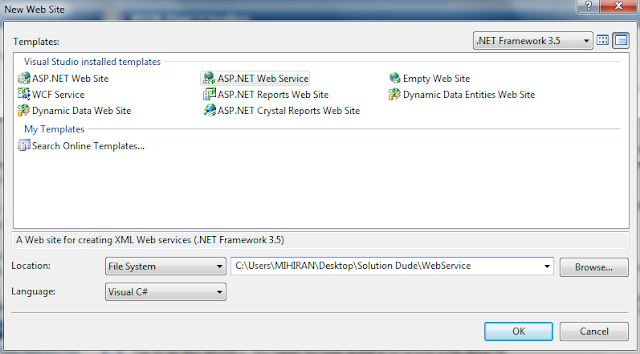
Now you have to modify connection string in your web.config file according to your configurations.
My one is shown below.
<connectionStrings>
<add name="ConStrine"
connectionString="Data Source=MIHIRAN-PC;
Initial Catalog=SolutionDude;
Trusted_Connection=true;
User ID=; Password="
providerName="System.Data.Sg1Client"/>
</connectionStrings>
Now I add a class called Member to the project. And I add a method called member which accept two parameters (name and password) and return a string value (id). What i'm going to do actually is when user submit the user name and the password, if he is a valid user he can enter the application. Now I modify that method I created like shown in below to connect and retrieve the values from SQL database.
Now i'm going to modify my Service.cs (default class) file like shown in below. memberValidation method will call the member class object and return id. If id have some value, then it is a valid member.
Now you have to do only one thing to publish the web service. It is attach the database to web service.
First go to sql server and detach your database. Then come to your web service project in visual studio and right click "App_Data" folder in solution explorer and select "Add existing item" and select your database file (with log file) My database files are stored in this location "C:\Program Files\Microsoft SQL Server\MSSQL10.MSSQLSERVER\MSSQL\DATA" .
After that go to SQL server again and attach the database to sql server.
OK. Now you are done with the web service. To test the web service run the Service.asmx file.
The method which I created in Service.cs file should be shown in top of the page.
Then click the method.
Then you can see the parameters of our memberValidation method are waiting for inputs. Give a valid user name and a password. According to my database I'm giving "mihiran" as user name and "123" as password.
And press "Invoke". If everything is fine you should be able to see a xml file holding your return value (id) like shown below.
Now you are done with the Web service.
But we we have another part. That is connecting the android application with this web service.
We'll see it in next chapter.
Thank you for the tutorial, but I am having trouble. Does the Member class go into the ISerivce file? Where does the member method go then?
ReplyDeletewhy is your username and password empty?
ReplyDeleteIn here, I'm checking the username and passwd by giving them. Those are not empty. According to my code if the username and passwd are correct then it returns id. (inside "member(username,passwd)" method)
Deletei made web services and also succesfully run web services after i have also made the Android Program but that is not working .
ReplyDeleteso plz help me
thanks in advance
my code is this in which implemented ksoap jar
//
package com.example.webservicetest;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapPrimitive;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends Activity {
EditText name,pass;
TextView tv;
private static final String SOAP_ACTION="http://tempuri.org/memberValidation" ;
private static final String METHOD_NAME="memberValidation";
private static final String NAMESPACE="http://tempuri.org/";
private static final String URL="http://10.0.2.2:1147/Websevice/Service.asmx";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
name=(EditText)findViewById(R.id.editText1);
pass=(EditText)findViewById(R.id.editText2);
tv=(TextView) findViewById(R.id.textView1);
}
public void onClick(View view)
{
SoapObject Request=new SoapObject(NAMESPACE,METHOD_NAME);
Request.addProperty("name",name.getText().toString());
Request.addProperty("password",pass.getText().toString());
SoapSerializationEnvelope sse;
sse=new SoapSerializationEnvelope(SoapEnvelope.VER11);
sse.dotNet=true;
sse.setOutputSoapObject(Request);
HttpTransportSE htp=new HttpTransportSE(URL);
try
{
htp.call(SOAP_ACTION,sse);
SoapPrimitive rs=(SoapPrimitive)sse.getResponse();
tv.setText("Login Successful");
}
catch(Exception e)
{
tv.setText("Login UnSuccessful");
}
}
}
according to this line "private static final String URL="http://10.0.2.2:1147/Websevice/Service.asmx" your port number of the web service should be "1147". please check the port number of your web service. if it is not 1147 please change it to 1147 and run again. set "Use dynamic ports = false" then set the port number. otherwise port number will be changed time to time..
Deletefor more info refer part 2. (http://androidplusiphone.blogspot.com/2012/03/android-chapter-4-web-services-in_27.html)
MY jar name is ksoap2.jar
ReplyDeletereally a great tutorial bro :) thanks alot
ReplyDeletejust one thing i need to know
what if i want to use real website to host that webservice instead of local host
what should i change in the code ?
Thx in advance
I never done it before dude. I think you have to change the "private static final String URL" string..
Delete:)
yeah it worked :D
Deletethanks alot ;)
Great !
DeleteThis comment has been removed by the author.
ReplyDeleteHi Mihirian! Thanks a lot for this tutorial, it works for me.
ReplyDeleteCan I call a SQL procedure or a function with a service like this?
Thanks!
yes it should work..
Deletewhen i press invoke i find this
ReplyDeleteThis XML file does not appear to have any style information associated with it. The document tree is shown below.
i think that my problem in the connection to the data base
maybe in this
connectionString="Data Source=OUSSAMA-PC; Initial Catalog=SolutionDude; Trusted_Connecion=true; User ID=;Password=" providerName="System.Data.SqlClient
what is your database name and server name? and please tell me what you are trying to do? what is invoke button?
Deletei try to do the same example like you i create my database name is SolustionDude located in OUSSAMA-PC\SQLEXPRESS but how can i know my server name.
ReplyDeletemy problem is when i press "invoke" after i put name and password, i didn't have the same result like you in the photo number 9.
sorry for my bad English.
i thanks a lot for your help.
ok. Change "Source=OUSSAMA-PC" to "Source=OUSSAMA-PC\SQLEXPRESS". If you use sql express edition you have to use like this.
DeleteAnother thing.. Did you add your activity to manifest file? If not see chapter one >> http://androidplusiphone.blogspot.com/2012/03/android-chapter-1-creating-layouts.html Thanks.
Deletethis the web service maybe i do a mistake :
Deletehttp://www.4shared.com/rar/FZ_Tw8ho/WebSite2.html
and my problem is in testing the web services hi didn't give me the id when i put a correct name and password.
Ok I'll check and let you know..
DeleteYou sent me only the solution file. please send me the whole project (webservice only)
Deletehttp://www.4shared.com/zip/dLknETpG/WebSite2.html
Deletei export the webservice
This comment has been removed by the author.
ReplyDeleteI think you created a wrong project. It should be ASP.NET Web Service. In VS 2010 we cannot find this option. Are you using VS 2010 or 2008? Anyway use and modify this. http://www.mediafire.com/download/42ugx24bhclgydu/WebSite1.rar
Deleteplease check and tell me whether it works or not. thanks.
thanks, but i didn't find the solution to open it i find only web.config service.asmx app_Code and app_Data.
Deleteplease can you send me the web service that you used in this tutorial
Hi Mihiran
ReplyDeleteI want to create the same thing but using the wcf to connect to android
basically a client and server relationship
could you post a tutorial for this or how would I go about accomplishing this connection?
I have tried other tutorials but they are always hallways
I am able to create the wcf in visual studios 2010 ultimate but when I try to link it to Android I always get errors
Thanks
Hi Mihiran.
ReplyDeleteI created in Visual studio 2012 WCF web service. but I can't connect to Android. I got error about "XMLpullparserException" . my service localhost adress is "http//..../service.svc" not asmx? What should I do? I used ksoap also.